Integrating AI into React Applications: A Practical Guide
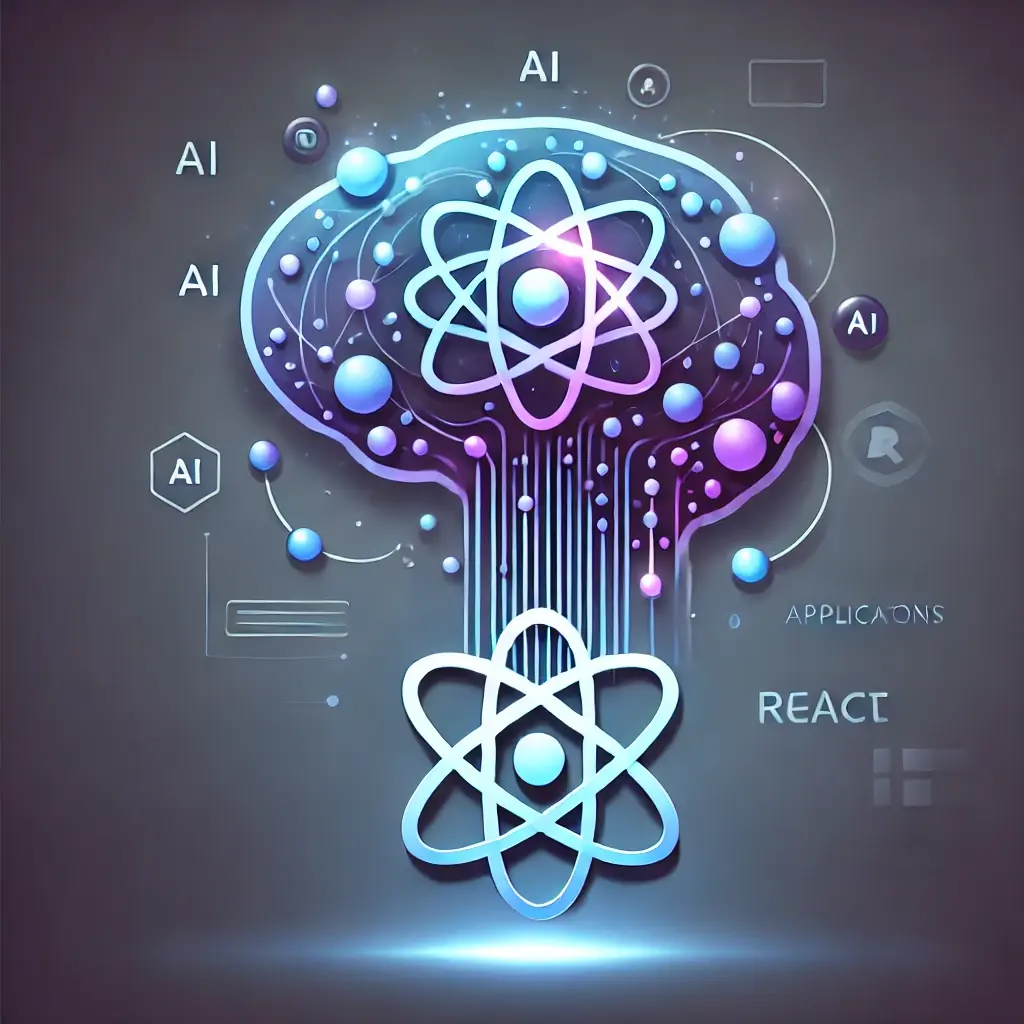
Artificial Intelligence is transforming the way we build web applications. For React developers, the integration of AI capabilities opens up endless possibilities for creating smarter, more responsive applications. This comprehensive guide will walk you through various AI integration methods, complete with practical examples and best practices.
Understanding AI Integration Methods
1. Natural Language Processing (NLP)
NLP enables applications to understand, interpret, and generate human language. Here's a detailed look at implementing different NLP features:
Chatbot Implementation
import { Configuration, OpenAIApi } from 'openai';
const ChatbotComponent = () => {
const [messages, setMessages] = useState([]);
const [isProcessing, setIsProcessing] = useState(false);
const processUserInput = async (userInput) => {
setIsProcessing(true);
const configuration = new Configuration({
apiKey: process.env.REACT_APP_OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
try {
const completion = await openai.createChatCompletion({
model: "gpt-3.5-turbo",
messages: [
{ role: "system", content: "You are a helpful assistant." },
...messages,
{ role: "user", content: userInput }
],
temperature: 0.7,
max_tokens: 150
});
const newMessage = completion.data.choices[0].message;
setMessages(prev => [...prev,
{ role: "user", content: userInput },
{ role: "assistant", content: newMessage.content }
]);
} catch (error) {
console.error('Error:', error);
} finally {
setIsProcessing(false);
}
};
return (
<div className="chatbot-container">
{/* Render chat messages */}
<div className="messages">
{messages.map((msg, index) => (
<div key={index} className={`message ${msg.role}`}>
{msg.content}
</div>
))}
</div>
{/* Input form */}
<ChatInputForm
onSubmit={processUserInput}
isProcessing={isProcessing}
/>
</div>
);
};
Text Analysis and Sentiment Detection
import { SentimentAnalyzer } from 'natural';
import aposToLexForm from 'apos-to-lex-form';
import SpellCorrector from 'spelling-corrector';
const TextAnalysisComponent = () => {
const [sentiment, setSentiment] = useState(null);
const spellCorrector = new SpellCorrector();
spellCorrector.loadDictionary();
const analyzeSentiment = (text) => {
// Pre-process text
const lexedText = aposToLexForm(text);
const casedText = lexedText.toLowerCase();
const alphaOnlyText = casedText.replace(/[^a-zA-Z\s]+/g, '');
// Correct spelling
const words = alphaOnlyText.split(' ').map(word =>
spellCorrector.correct(word)
);
const analyzer = new SentimentAnalyzer('English', 'afinn');
const result = analyzer.getSentiment(words);
return {
score: result,
label: result > 0 ? 'Positive' : result < 0 ? 'Negative' : 'Neutral',
confidence: Math.abs(result) / 5 // Normalize to 0-1 range
};
};
// Component implementation
};
2. Computer Vision Integration
Computer vision allows applications to understand and process visual information. Here's a detailed implementation using TensorFlow.js:
Image Classification System
import * as tf from '@tensorflow/tfjs';
import * as mobilenet from '@tensorflow-models/mobilenet';
const ImageClassificationComponent = () => {
const [model, setModel] = useState(null);
const [predictions, setPredictions] = useState([]);
const [isLoading, setIsLoading] = useState(true);
useEffect(() => {
loadModel();
}, []);
const loadModel = async () => {
try {
const loadedModel = await mobilenet.load({
version: 2,
alpha: 1.0
});
setModel(loadedModel);
setIsLoading(false);
} catch (error) {
console.error('Error loading model:', error);
}
};
const classifyImage = async (imageElement) => {
if (!model) return;
try {
const predictions = await model.classify(imageElement, 5);
setPredictions(predictions.map(pred => ({
className: pred.className,
probability: (pred.probability * 100).toFixed(2)
})));
} catch (error) {
console.error('Error classifying image:', error);
}
};
return (
<div className="image-classification">
{isLoading ? (
<LoadingSpinner />
) : (
<>
<ImageUploader onImageLoad={classifyImage} />
<PredictionResults predictions={predictions} />
</>
)}
</div>
);
};
Business Benefits
1. Enhanced User Experience
- Personalized content recommendations
- Intelligent search capabilities
- Real-time assistance through chatbots
2. Improved Decision Making
- Data-driven insights
- Predictive analytics
- User behavior analysis
3. Automation
- Automated content moderation
- Smart form filling
- Document processing
Conclusion
Integrating AI into React applications can significantly enhance their capabilities and user experience. By choosing the right tools and following best practices, you can create intelligent applications that provide real value to your users.
Remember to:
- Start with clear use cases
- Choose appropriate AI services
- Implement proper error handling
- Monitor performance and costs
- Keep security in mind
The future of web applications lies in intelligent features, and React provides an excellent platform for implementing these capabilities.