Implementing NFC in React Native: A Complete Guide
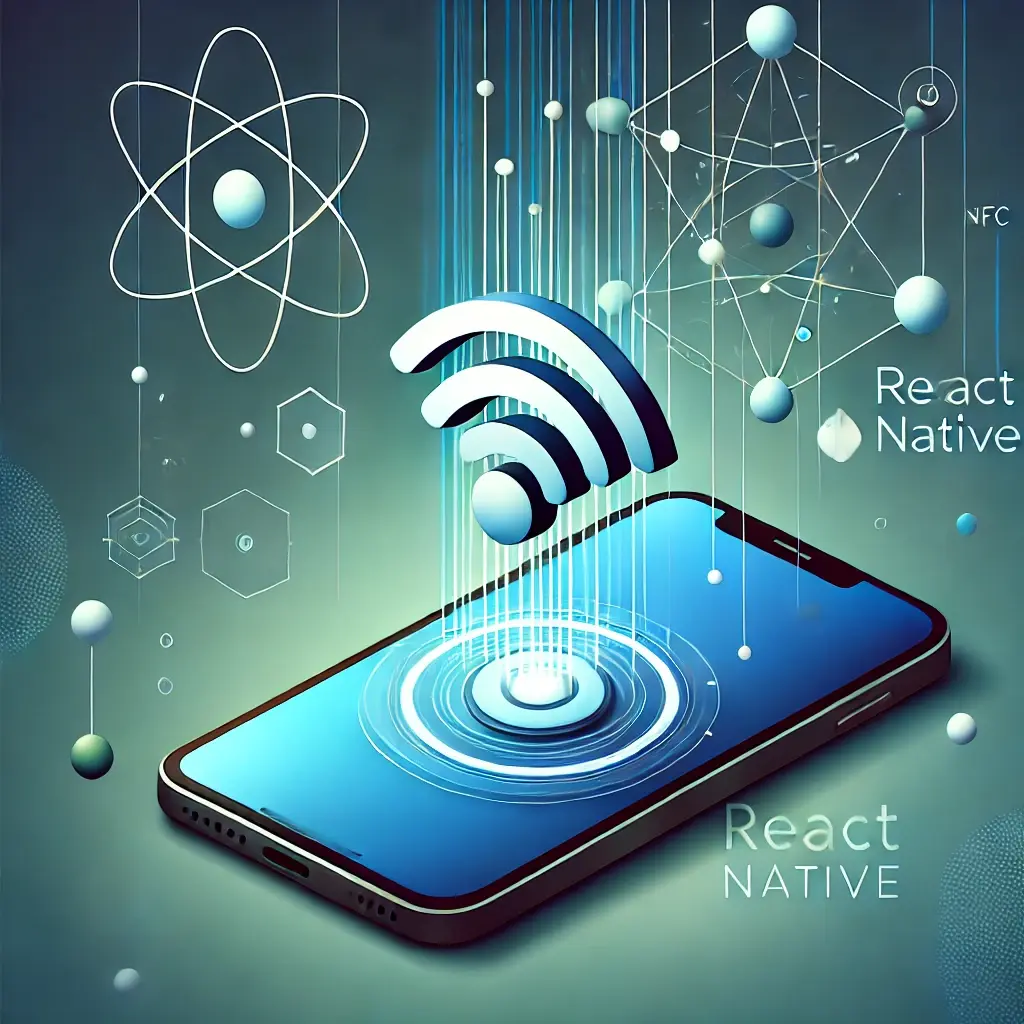
NFC (Near Field Communication) technology enables short-range wireless communication between devices. In this guide, we'll walk through implementing NFC functionality in a React Native application.
Prerequisites
- React Native development environment setup
- A physical Android/iOS device with NFC capabilities
- Basic understanding of React Native
Installation
First, install the required dependencies:
npm install react-native-nfc-manager
# or
yarn add react-native-nfc-manager
For iOS, add the following to your Info.plist
:
<key>NFCReaderUsageDescription</key>
<string>We need to use NFC</string>
<key>com.apple.developer.nfc.readersession.formats</key>
<array>
<string>NDEF</string>
<string>TAG</string>
</array>
Basic Implementation
Here's a simple example of how to implement NFC reading functionality:
import React, { useState, useEffect } from 'react';
import {
View,
Text,
Button,
Platform,
Alert,
} from 'react-native';
import NfcManager, { NfcTech } from 'react-native-nfc-manager';
const NFCScanner = () => {
const [isNfcSupported, setIsNfcSupported] = useState<boolean>(false);
useEffect(() => {
const checkNFCSupport = async () => {
const supported = await NfcManager.isSupported();
setIsNfcSupported(supported);
if (supported) {
await NfcManager.start();
}
};
checkNFCSupport();
return () => {
NfcManager.cancelTechnologyRequest();
};
}, []);
const readNFC = async () => {
try {
await NfcManager.requestTechnology(NfcTech.Ndef);
const tag = await NfcManager.getTag();
console.log('Tag found:', tag);
Alert.alert('Success', 'NFC Tag read successfully!');
} catch (error) {
console.warn('Error reading NFC:', error);
Alert.alert('Error', 'Failed to read NFC tag');
} finally {
NfcManager.cancelTechnologyRequest();
}
};
if (!isNfcSupported) {
return (
<View>
<Text>NFC not supported on this device</Text>
</View>
);
}
return (
<View>
<Button title="Scan NFC Tag" onPress={readNFC} />
</View>
);
};
export default NFCScanner;
Writing to NFC Tags
Here's how to write data to an NFC tag:
const writeNFC = async () => {
try {
await NfcManager.requestTechnology(NfcTech.Ndef);
const bytes = Ndef.encodeMessage([
Ndef.textRecord('Hello from React Native!'),
]);
if (bytes) {
await NfcManager.ndefHandler.writeNdefMessage(bytes);
Alert.alert('Success', 'Data written to NFC tag!');
}
} catch (error) {
console.warn('Error writing to NFC:', error);
Alert.alert('Error', 'Failed to write to NFC tag');
} finally {
NfcManager.cancelTechnologyRequest();
}
};
Best Practices
- Error Handling: Always implement proper error handling and cleanup:
const cleanupNFC = () => {
NfcManager.cancelTechnologyRequest().catch(() => {
// ignore cleanup errors
});
};
- Platform-Specific Code: Handle platform differences appropriately:
const initNFC = async () => {
if (Platform.OS === 'android') {
// Android-specific initialization
await NfcManager.requestTechnology(NfcTech.NfcA);
} else {
// iOS-specific initialization
await NfcManager.requestTechnology(NfcTech.NFCTagReaderSession);
}
};
- Background Handling: Properly handle NFC when app is in background:
useEffect(() => {
AppState.addEventListener('change', handleAppStateChange);
return () => {
AppState.removeEventListener('change', handleAppStateChange);
};
}, []);
const handleAppStateChange = (nextAppState: string) => {
if (nextAppState === 'background') {
cleanupNFC();
}
};
Common Issues and Solutions
1. NFC Not Detecting Tags
Make sure:
- NFC is enabled in device settings
- The tag is properly positioned
- You're using the correct NFC technology type
2. iOS Specific Requirements
For iOS, you need:
- A proper provisioning profile with NFC capabilities
- The correct entitlements in your project
- iOS 13 or later for background tag reading
Conclusion
Implementing NFC in React Native opens up many possibilities for creating interactive mobile applications. While the initial setup might seem complex, the react-native-nfc-manager library makes it straightforward to add NFC capabilities to your app.
Remember to:
- Always handle errors appropriately
- Clean up NFC resources when not in use
- Test thoroughly on both Android and iOS devices
- Consider platform-specific differences in implementation